Golang How to Return Multiple Value and Error Handling
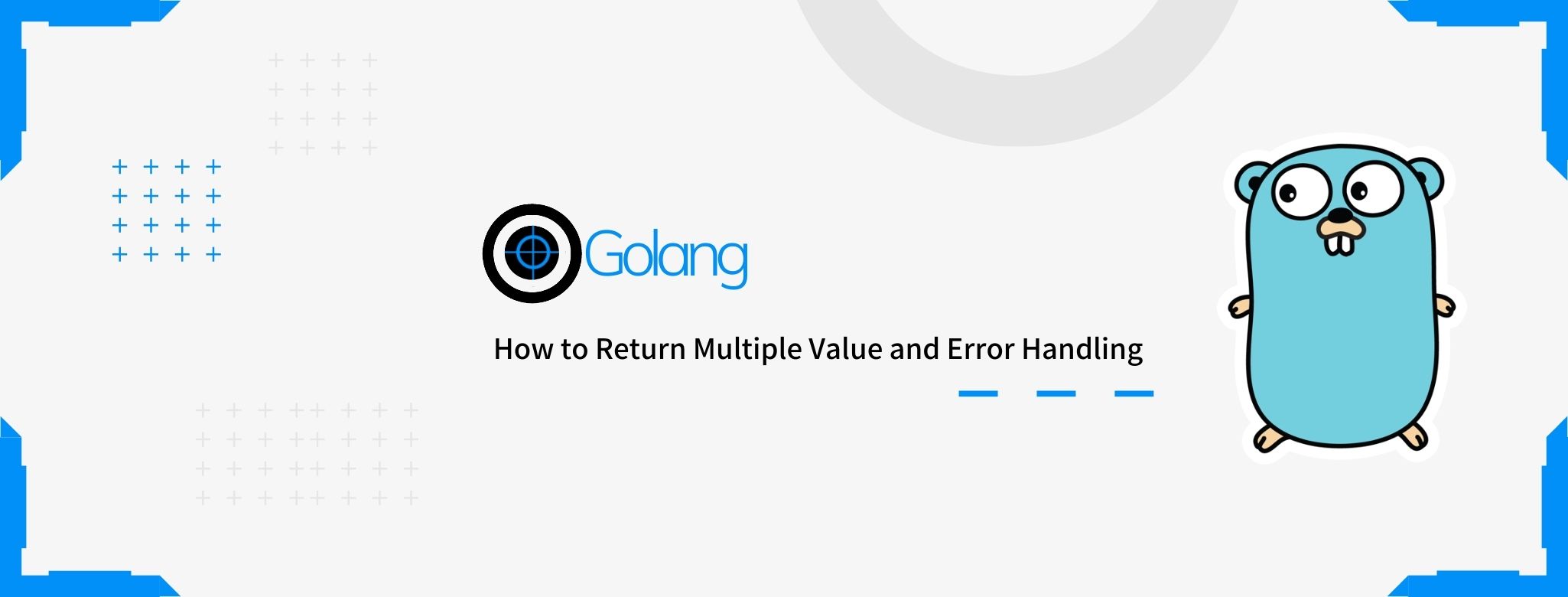
Contents
Go has built-in support to return more than one values and its main use case is to identify any error.
Synopsis
We have used In most programming languages, functions can return single value, but in Go, they can return any number of values.
The main use case of returning more than one values is to return the functions result and then the value to identify any error.
Example:1 Function returns result and error
The below function shows that the function returns result and then the value to identify any error
|
|
Example:2 Function return multiple value
The below function shows that it returns 2 ints.
|
|